How To Generate A Sitemap In Laravel Using Spatie Laravel Sitemap Package
Published on October 16, 2024 by Dinesh Uprety
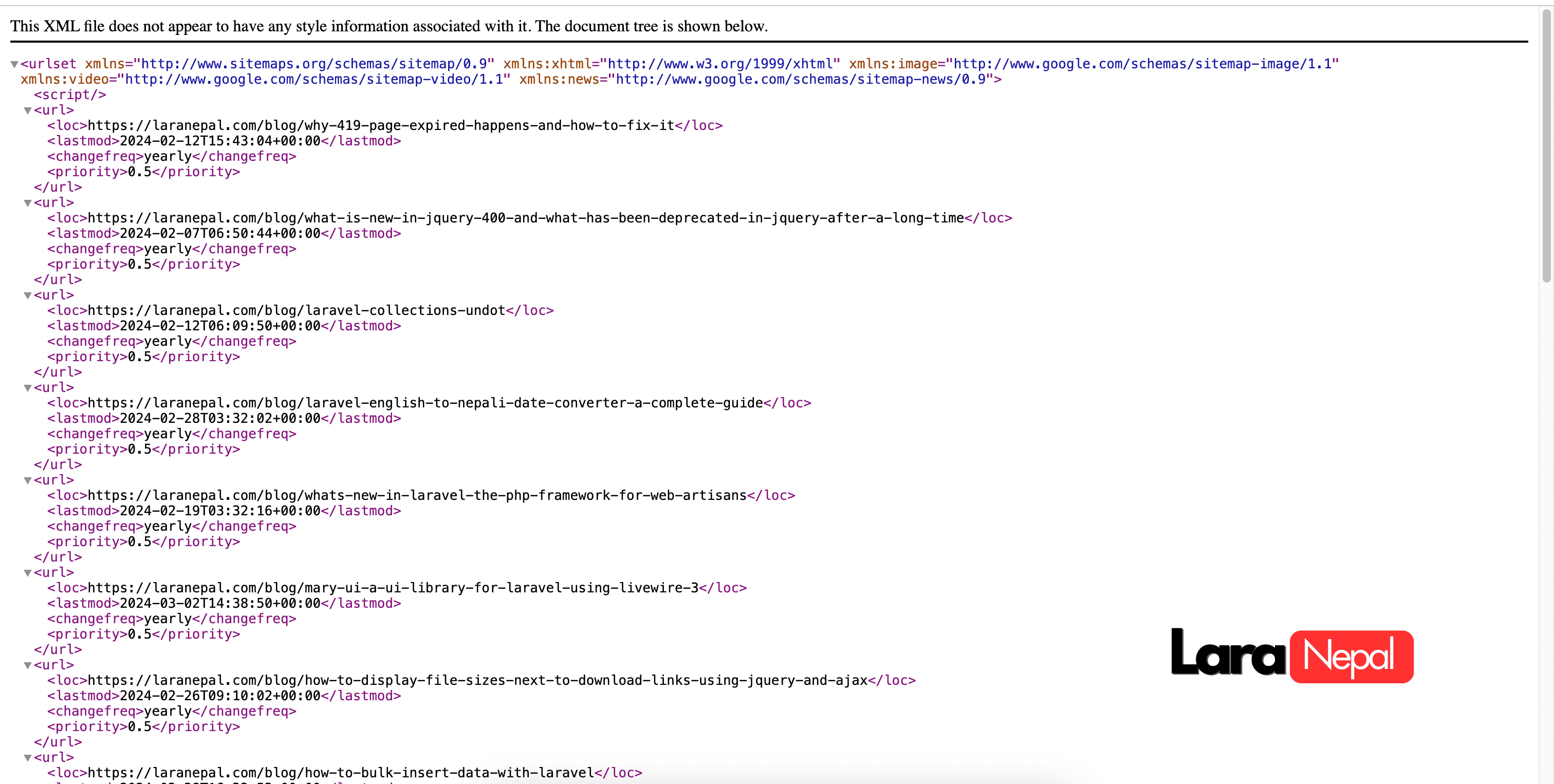
Step-by-Step Guide to Generating a Sitemap in Laravel with Spatie
Creating a sitemap is essential for improving your website's SEO
and ensuring that search engines can easily index
your
content. In this article, we'll walk you through the steps to generate a sitemap for your Laravel application using the
Spatie Laravel Sitemap package.
Step 1: Install the Spatie Laravel Sitemap Package
To get started, you need to install the Spatie Laravel Sitemap package. You can do this by running the following command:
composer require spatie/laravel-sitemap
Step 2: Publish the Configuration File
Next, you need to publish the configuration file for the package. You can do this by running the following command:
php artisan vendor:publish --provider="Spatie\Sitemap\SitemapServiceProvider" --tag=config
Step 3: Create a Sitemap Generation Class
To generate a sitemap, you need to create a class that extends the Spatie\Sitemap\Sitemap
class. This class
will be responsible for generating the sitemap content.
In this example, we'll create a class called BuildSitemap in the App\Actions
namespace:
<?php namespace App\Actions; use App\Models\Category;use App\Models\Post;use Spatie\Sitemap\Sitemap;use Spatie\Sitemap\Tags\Url; class BuildSitemap{ public function build(): void { Sitemap::create() ->add($this->build_index(Post::published() ->visible() ->get(), 'sitemap_articles.xml')) ->add($this->build_index(Category::visible()->get(), 'sitemap_categories.xml')) ->add(Url::create('/')->setPriority(1)->setChangeFrequency(URL::CHANGE_FREQUENCY_ALWAYS)) ->add(Url::create('/newsletter')->setPriority(0.5)->setChangeFrequency(URL::CHANGE_FREQUENCY_MONTHLY)) ->add(Url::create('/about')->setPriority(0.5)->setChangeFrequency(URL::CHANGE_FREQUENCY_MONTHLY)) ->add(Url::create('/contact')->setPriority(0.5)->setChangeFrequency(URL::CHANGE_FREQUENCY_MONTHLY)) ->add(Url::create('/ads')->setPriority(0.5)->setChangeFrequency(URL::CHANGE_FREQUENCY_MONTHLY)) ->add(Url::create('/aboutme')->setPriority(0.5)->setChangeFrequency(URL::CHANGE_FREQUENCY_MONTHLY)) ->writeToFile(public_path('sitemap.xml')); } protected function build_index($model, $path): string { Sitemap::create() ->add($model) ->writeToFile(public_path($path)); return $path; }}
note: This example was from my personal project, you can customize it to fit your needs. you can add more routes to the sitemap. you can also add more models to the sitemap. i have added a sitemap for articles and categories.
Step 4: Run the Sitemap Generation
To generate the sitemap, you need to call the build
method on the BuildSitemap
class. You can do this from a
controller, a command, or any other part of your application where you want to trigger the sitemap generation.
For example, you can create an Artisan command to generate the sitemap:
<?php namespace App\Console\Commands; use App\Actions\BuildSitemap;use Illuminate\Console\Command; class GenerateSitemap extends Command{ protected $signature = 'sitemap:generate'; protected $description = 'Generate the sitemap for the application'; public function handle(BuildSitemap $buildSitemap): int { $buildSitemap->build(); $this->info('Sitemap generated successfully.'); return 0; }}
You can then run the command to generate the sitemap:
php artisan sitemap:generate
Conclusion
Generating a sitemap for your Laravel application is essential for improving your website's SEO and ensuring that search engines can easily index your content. By following the steps outlined in this article, you can create a sitemap for your application using the Spatie Laravel Sitemap package.
I hope you found this article helpful. If you have any questions or feedback, feel free to leave a comment below.
Happy coding! 🚀